Laravel Collections: Tips and Tricks
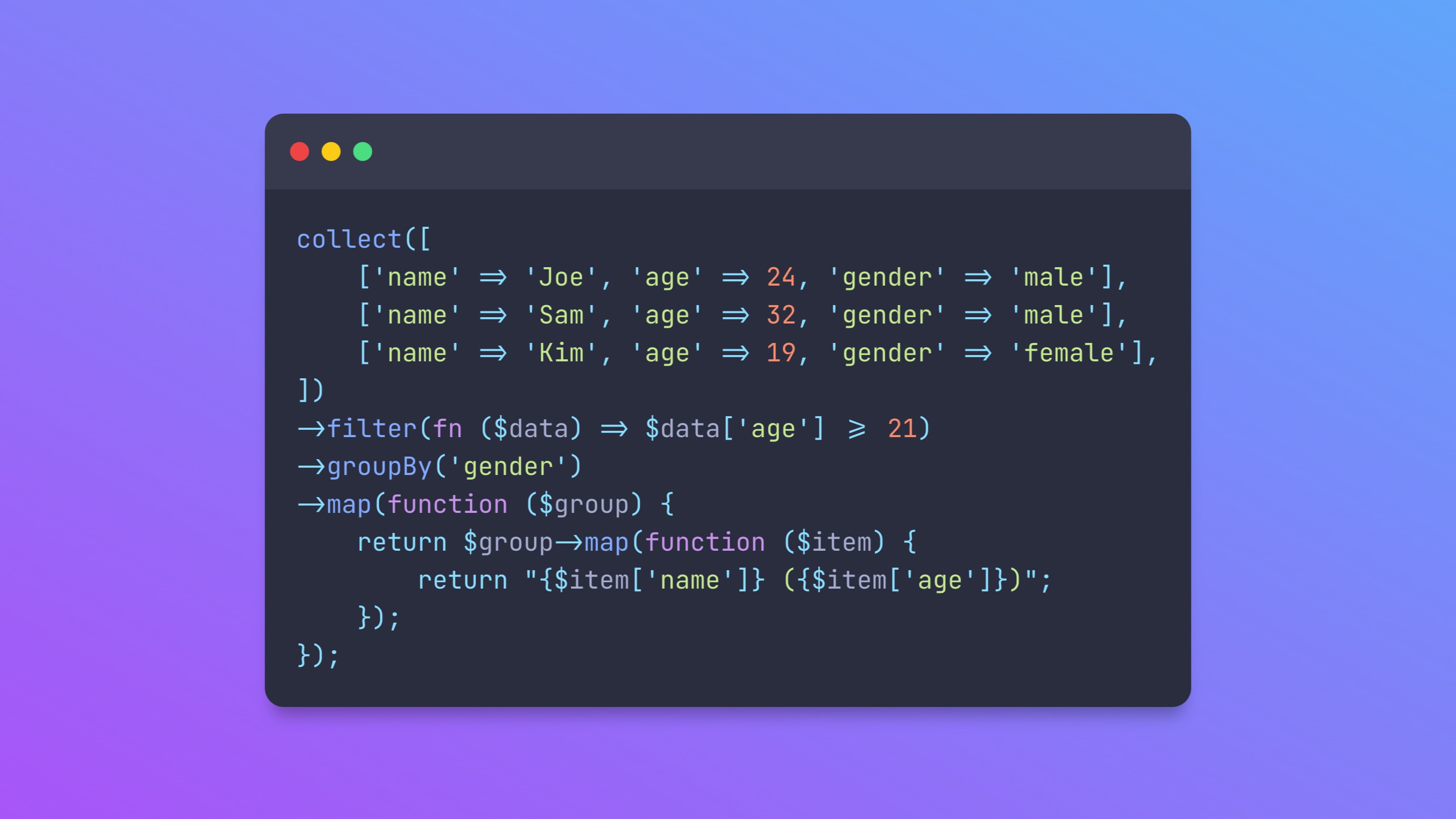
- Creating a Collection
- Filtering Data
- Mapping Data
- Sorting Data
- Chaining Methods
- Grouping Data
- Flattening Data
- Reducing Data
- Transforming Data
- Custom Sorting
- Filtering by Multiple Criteria
- Checking for Existence
- Summing Values
- Chunks
- cursor() method
- chunk() vs cursor()
Laravel is a popular PHP framework known for its expressive and elegant syntax. One of the many features that make Laravel so popular is its collections. Collections are a powerful tool for working with arrays of data. They provide a fluent, chainable API for filtering, sorting, and manipulating data.
In this blog post, we'll explore some of the most useful tips and tricks for working with Laravel collections. We'll provide practical code examples that you can use to improve your own code.
Creating a Collection
Creating a collection in Laravel is easy. You can use the collect helper function to create a new collection from an array.
$items = collect([1, 2, 3, 4, 5]);
You can also create a collection from an associative array.
$data = [
['name' => 'John', 'age' => 21],
['name' => 'Jane', 'age' => 25],
['name' => 'Bob', 'age' => 30],
];
$users = collect($data);
Filtering Data
Collections make it easy to filter data. You can use the filter method to create a new collection containing only the items that pass a given truth test.
$items = collect([1, 2, 3, 4, 5]);
$filtered = $items->filter(function ($item) {
return $item > 3;
});
// Output: [4, 5]
You can also use the where method to filter a collection by a key-value pair.
$users = collect([
['name' => 'John', 'age' => 21],
['name' => 'Jane', 'age' => 25],
['name' => 'Bob', 'age' => 30],
]);
$filtered = $users->where('age', '>', 25);
// Output: [['name' => 'Bob', 'age' => 30]]
Mapping Data
Collections also make it easy to map data. You can use the map method to create a new collection containing the results of applying a callback function to each item in the original collection.
$items = collect([1, 2, 3, 4, 5]);
$mapped = $items->map(function ($item) {
return $item * 2;
});
// Output: [2, 4, 6, 8, 10]
You can also use the pluck method to retrieve a single column from a collection.
$users = collect([
['name' => 'John', 'age' => 21],
['name' => 'Jane', 'age' => 25],
['name' => 'Bob', 'age' => 30],
]);
$names = $users->pluck('name');
// Output: ['John', 'Jane', 'Bob']
Sorting Data
Collections make it easy to sort data. You can use the sort method to sort a collection in ascending order.
$items = collect([3, 2, 1, 5, 4]);
$sorted = $items->sort();
// Output: [1, 2, 3, 4, 5]
Chaining Methods
One of the most powerful features of Laravel collections is the ability to chain methods together. This allows you to perform multiple operations on a collection in a single line of code. For example, you can filter a collection, map the remaining items, and then sort the results:
$items = collect([3, 2, 1, 5, 4]);
$sorted = $items
->filter(function ($item) {
return $item > 3;
})
->map(function ($item) {
return $item * 2;
})
->sort();
// Output: [8, 10]
Grouping Data
You can use the groupBy method to group a collection by a given key. This is useful when you have a collection of items and you want to group them by a certain attribute.
$users = collect([
['name' => 'John', 'age' => 21, 'gender' => 'male'],
['name' => 'Jane', 'age' => 25, 'gender' => 'female'],
['name' => 'Bob', 'age' => 30, 'gender' => 'male'],
['name' => 'Lisa', 'age' => 28, 'gender' => 'female'],
]);
$grouped = $users->groupBy('gender');
// Output:
// [
// 'male' => [
// ['name' => 'John', 'age' => 21, 'gender' => 'male'],
// ['name' => 'Bob', 'age' => 30, 'gender' => 'male'],
// ],
// 'female' => [
// ['name' => 'Jane', 'age' => 25, 'gender' => 'female'],
// ['name' => 'Lisa', 'age' => 28, 'gender' => 'female'],
// ],
// ]
Flattening Data
You can use the flatten method to flatten a multi-dimensional collection into a single level collection.
$collection = collect([
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
]);
$flattened = $collection->flatten();
// Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
Reducing Data
You can use the reduce method to reduce a collection to a single value. This is useful when you have a collection of items and you want to perform a calculation on them.
$collection = collect([1, 2, 3, 4, 5]);
$total = $collection->reduce(function ($carry, $item) {
return $carry + $item;
});
// Output: 15
Transforming Data
You can use the transform method to modify a collection in place. This is useful when you want to modify the items in a collection without creating a new collection.
$users = collect([
['name' => 'John', 'age' => 21],
['name' => 'Jane', 'age' => 25],
['name' => 'Bob', 'age' => 30],
]);
$users->transform(function ($user) {
// Add a new "isAdult" property to the user based on their age
$user['isAdult'] = $user['age'] >= 18;
// Capitalize the user's name
$user['name'] = ucwords($user['name']);
return $user;
});
// Output:
// [
// ['name' => 'John', 'age' => 21, 'isAdult' => true],
// ['name' => 'Jane', 'age' => 25, 'isAdult' => true],
// ['name' => 'Bob', 'age' => 30, 'isAdult' => true],
// ]
Custom Sorting
You can use the sortBy method to sort a collection based on a given key. If you need to perform custom sorting, you can use the sortByDesc method and pass a closure as an argument. The closure should return a numeric value that will be used for comparison.
$items = collect([
['name' => 'John', 'age' => 21],
['name' => 'Jane', 'age' => 25],
['name' => 'Bob', 'age' => 30],
]);
$sorted = $items->sortByDesc(function ($item) {
return $item['age'];
});
// Output:
// [
// ['name' => 'Bob', 'age' => 30],
// ['name' => 'Jane', 'age' => 25],
// ['name' => 'John', 'age' => 21],
// ]
Filtering by Multiple Criteria
You can use the filter method to filter a collection based on a single criteria. If you need to filter by multiple criteria, you can chain multiple filter calls together.
$users = collect([
['name' => 'John', 'age' => 21, 'gender' => 'male'],
['name' => 'Jane', 'age' => 25, 'gender' => 'female'],
['name' => 'Bob', 'age' => 30, 'gender' => 'male'],
['name' => 'Lisa', 'age' => 28, 'gender' => 'female'],
]);
$filtered = $users->filter(function ($user) {
return $user['gender'] == 'female' && $user['age'] > 25;
});
// Output:
// [
// ['name' => 'Lisa', 'age' => 28, 'gender' => 'female'],
// ]
Checking for Existence
You can use the contains method to check if a collection contains a specific value. If you need to check for multiple values, you can pass an array of values to the contains method.
$collection = collect([1, 2, 3, 4, 5]);
$hasThree = $collection->contains(3); // true
$hasSix = $collection->contains(6); // false
$hasTwoOrFive = $collection->contains([2, 5]); // true
$hasTwoOrSix = $collection->contains([2, 6]); // false
Summing Values
You can use the sum method to sum the values in a collection.
$collection = collect([1, 2, 3, 4, 5]);
$total = $collection->sum(); // 15
Chunks
In this example, we create a collection of user data. We then use the chunk method to split the collection into chunks of three items each.
$collection = collect([
['name' => 'John', 'age' => 21],
['name' => 'Jane', 'age' => 25],
['name' => 'Bob', 'age' => 30],
['name' => 'Lisa', 'age' => 28],
['name' => 'Steve', 'age' => 35],
['name' => 'Mary', 'age' => 29],
['name' => 'Joe', 'age' => 27],
]);
$chunks = $collection->chunk(3);
// Output:
// [
// [
// ['name' => 'John', 'age' => 21],
// ['name' => 'Jane', 'age' => 25],
// ['name' => 'Bob', 'age' => 30],
// ],
// [
// ['name' => 'Lisa', 'age' => 28],
// ['name' => 'Steve', 'age' => 35],
// ['name' => 'Mary', 'age' => 29],
// ],
// [
// ['name' => 'Joe', 'age' => 27],
// ],
// ]
The resulting $chunks
variable is a new collection of collections, with each sub-collection containing three items. The last sub-collection may contain fewer than three items if the original collection's length is not evenly divisible by three.
You can use this technique to process large collections in batches, or to paginate data in your application.
cursor()
method
// Example 1: Using cursor to process large data sets
$users = User::cursor();
foreach ($users as $user) {
// Do some processing on each user
// This will load each user one at a time into memory
}
// Example 2: Using cursor with filter to find the first matching record
$firstActiveUser = User::where('status', 'active')->cursor()->first();
// Example 3: Using cursor with filter and take to find the first N matching records
$activeUsers = User::where('status', 'active')->cursor()->take(10)->get();
In the first example, we use the cursor method to process a large data set of User records without loading all of the records into memory at once. Instead, each User is loaded one at a time, allowing you to process the data without consuming too much memory.
In the second example, we use the cursor method with a where filter to find the first User record with a status of "active". Because we are using a cursor, only the first matching record will be loaded into memory, even if there are thousands of active users in the database.
In the third example, we use the cursor method with a where filter and the take method to find the first 10 User records with a status of "active". Again, because we are using a cursor, only the first 10 matching records will be loaded into memory, even if there are thousands of active users in the database.
These examples demonstrate how the cursor method can be used to efficiently process large data sets without consuming too much memory. By loading data one record at a time, you can improve the performance and scalability of your application, especially when working with large amounts of data.
chunk()
vs cursor()
If you're working with large data sets in Laravel, you may have encountered memory or performance issues when loading your data into memory. Thankfully, Laravel Collections provides two methods that can help: cursor and chunk. But which one should you use?
In general, the cursor method is faster but uses more memory, while the chunk method uses a constant amount of memory but may be slower. But how much of a difference does it really make? Let's take a look at some benchmarks to find out.
We created a sample data set of 10,000 and 100,000 records and ran tests using each method. Here are the results:
Test Results for 10,000 Records
Method | Time (sec) | Memory (MB) |
---|---|---|
get() | 0.17 | 22 |
chunk(100) | 0.38 | 10 |
chunk(1000) | 0.17 | 12 |
cursor() | 0.16 | 14 |
As you can see, the cursor method is the fastest, with a run time of 0.16 seconds. However, it does use slightly more memory than the chunk methods.
Test Results for 100,000 Records
Method | Time (sec) | Memory (MB) |
---|---|---|
get() | 0.8 | 132 |
chunk(100) | 19.9 | 10 |
chunk(1000) | 2.3 | 12 |
chunk(10000) | 1.1 | 34 |
cursor() | 0.5 | 45 |
When we increase the number of records to 100,000, the differences become even more pronounced. The get method takes almost a second to run and consumes a lot of memory, while the chunk method using a chunk size of 100 takes nearly 20 seconds to run but uses very little memory. The cursor method is once again the fastest, taking only half a second to run, but it does use significantly more memory than the chunk methods.
So which method should you use? It depends on your specific use case. If you need the fastest possible performance and don't mind using a bit more memory, the cursor method is a great choice. If you need to conserve memory, especially when working with very large data sets, the chunk method may be a better option. Either way, you now have a better understanding of how these two methods work and how they can be used to process large data sets in Laravel.
In conclusion, the cursor and chunk methods in Laravel Collections provide powerful tools for processing large data sets efficiently. By understanding the performance characteristics of each method, you can make an informed decision about which one to use in your application. Whether you need the speed of the cursor method or the memory efficiency of the chunk method, Laravel Collections has you covered.
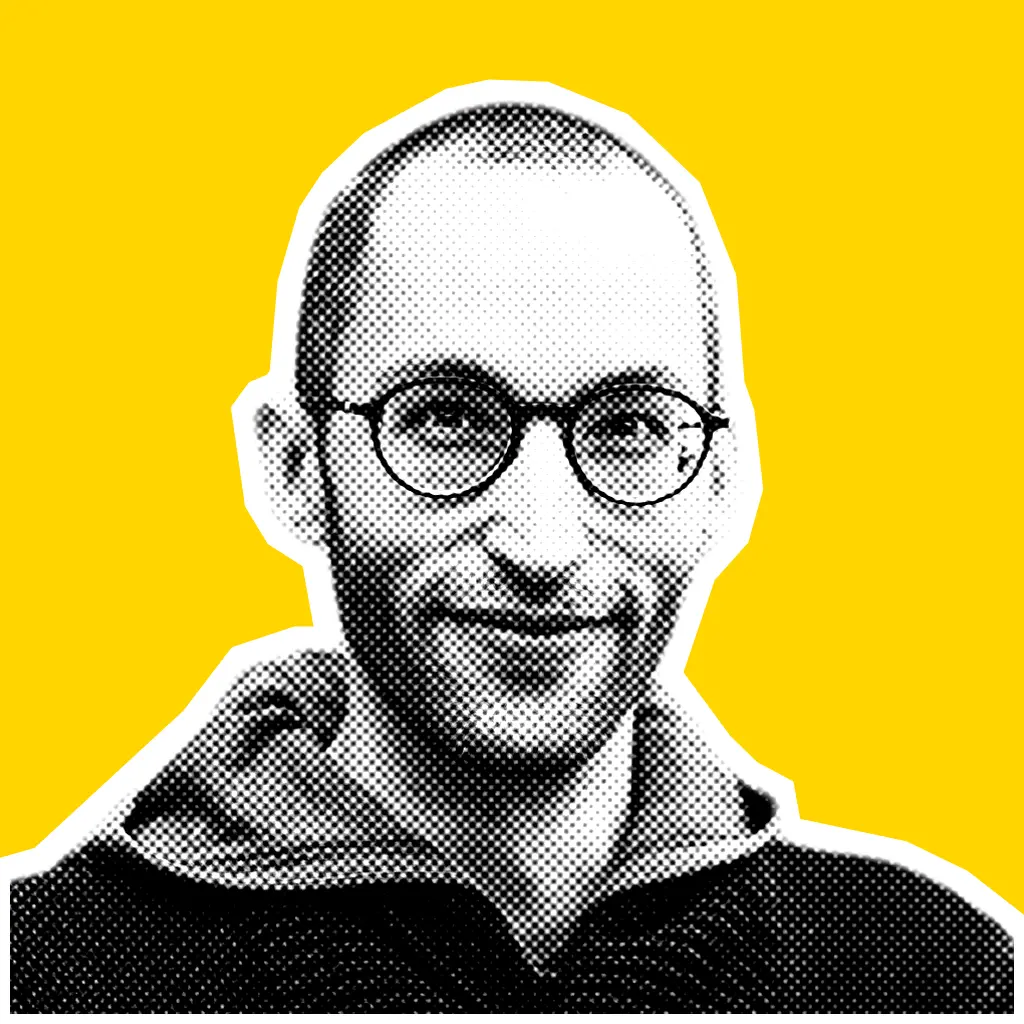
I'm a full-stack web developer working with the TALL stack.