How to Use Laravel Model Events on Pivot Tables
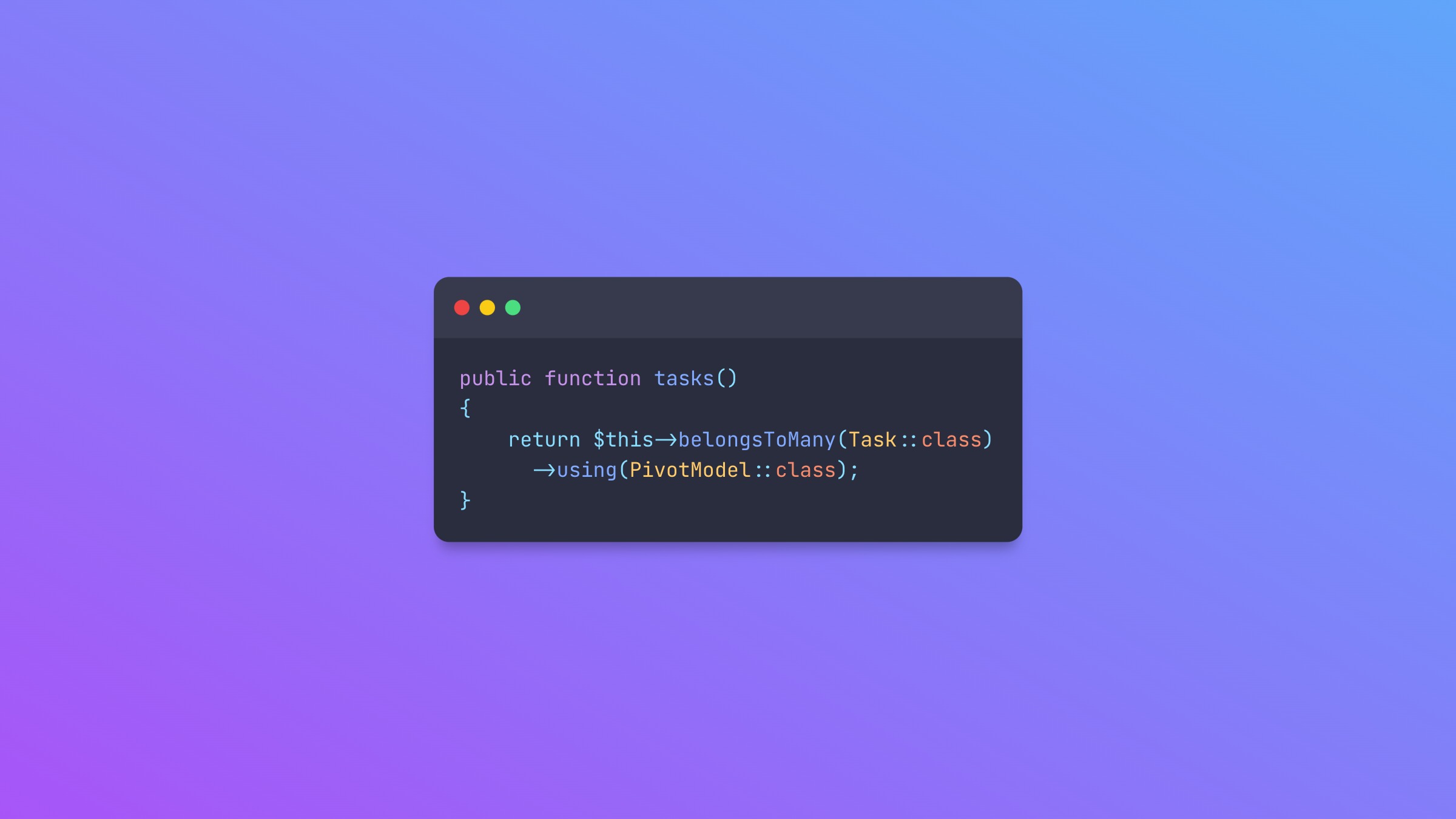
In a Laravel app, you may want to assign users
to tasks
while also tracking who assigned each user
to a task
. By utilizing Laravel's model events on pivot tables, this is easily achievable.
To begin, create three tables. One for tasks
, one for users
, and one for assigning users to tasks: task_user
. Then, create the corresponding TaskUser
pivot model using the following command:
php artisan make:model TaskUser --pivot
Next, add event handlers to the TaskUser
model by adding the following code:
class TaskUser extends Pivot
{
protected static function booted()
{
static::creating(function ($pivot_model) {
// ...
});
// + any other event handler
}
}
Now, it's important to let Laravel know which model it should use for the pivot table. This is achieved by adding the following code to the User model
:
public function tasks()
{
return $this->belongsToMany(Task::class)
->using(TaskUser::class);
}
Finally, you can assign users to tasks using the attach method on the user's tasks relationship, like so:
$user->tasks()->attach($tasks);
With these steps, your event callbacks or corresponding observer methods will be executed as expected. You now have a fully functional way to assign users to tasks while also tracking who assigned them.
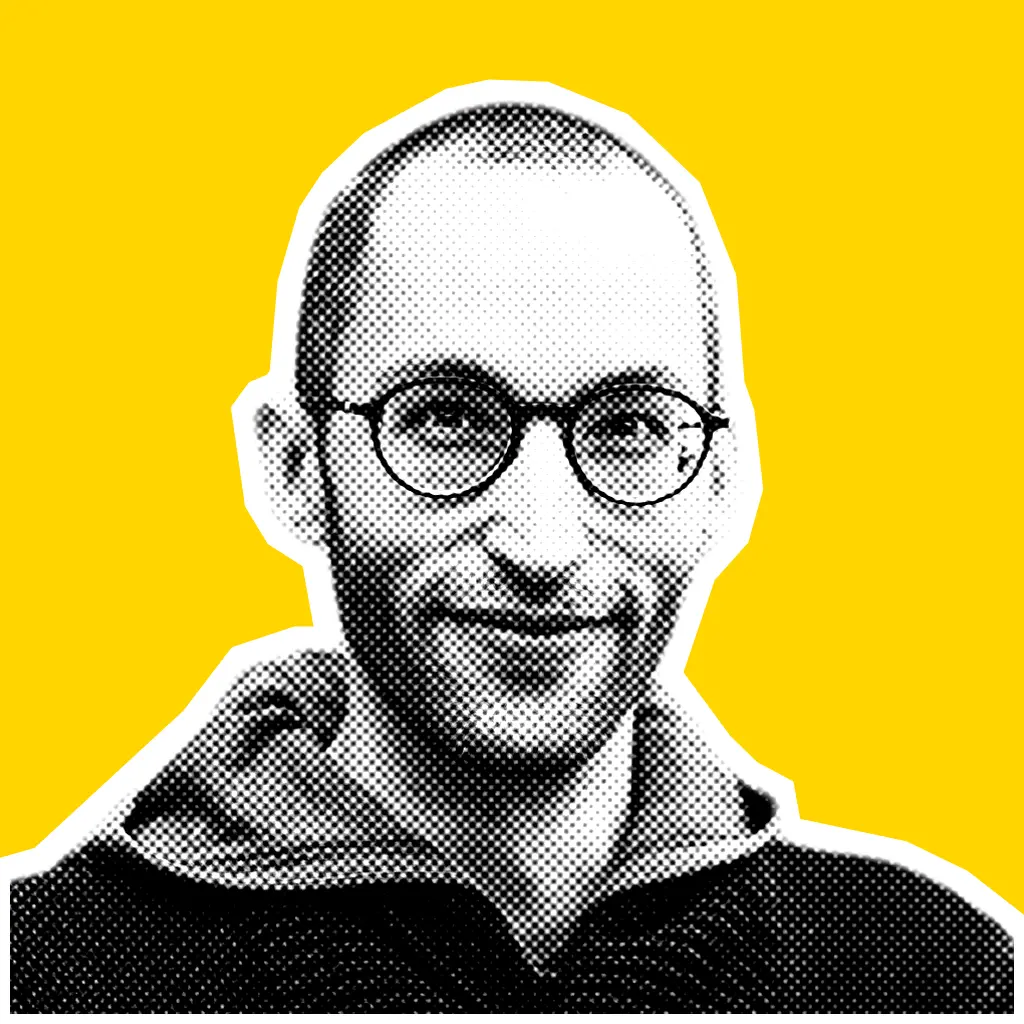
I'm a full-stack web developer working with the TALL stack.