Laravel JSON column management made easy with AsArrayObject cast
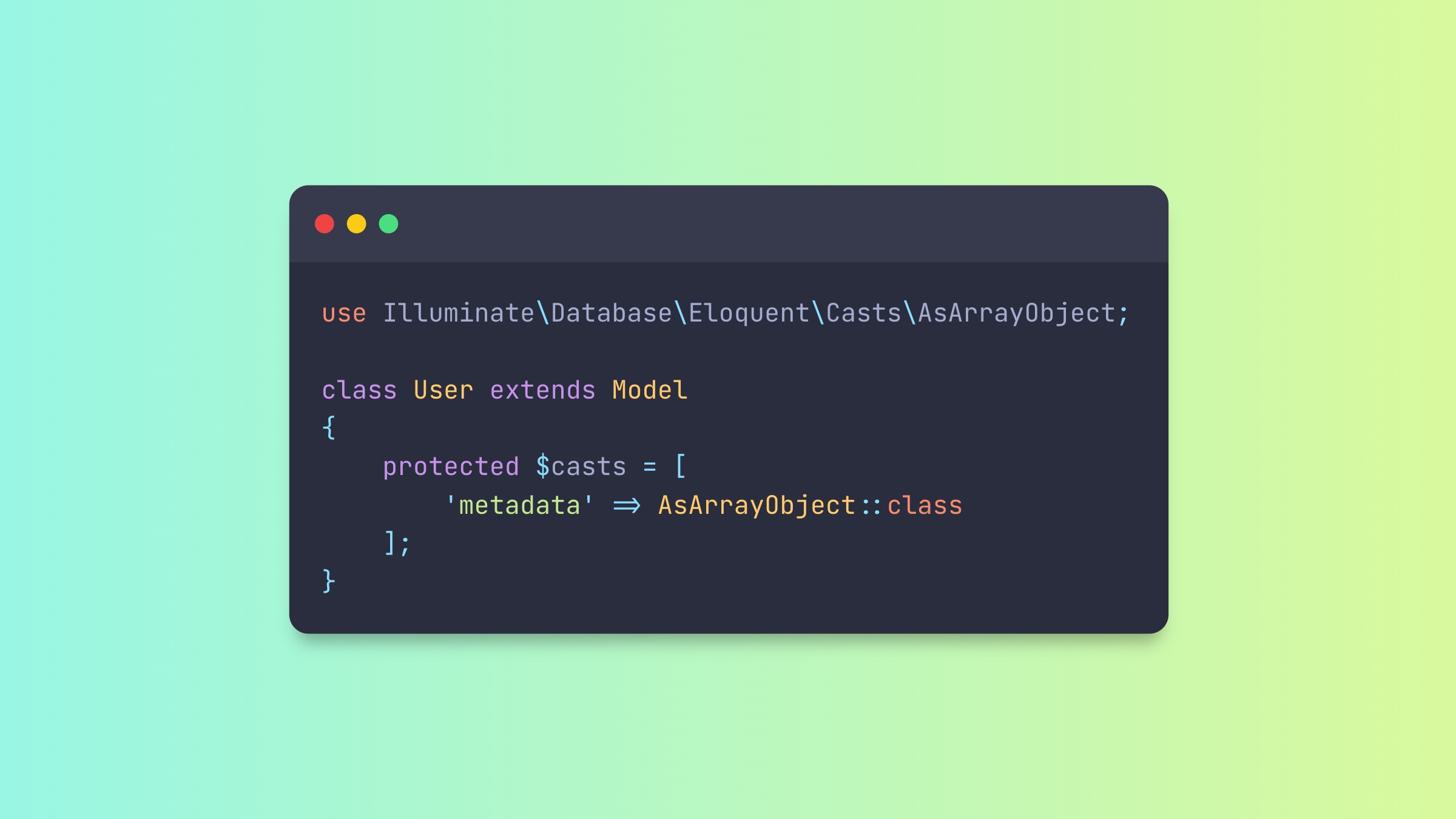
Hey there! 👋 If you're using Laravel to build your web applications, you might have come across JSON columns in your database tables. While they can be super useful for storing data, it can be a pain to update specific properties without changing the entire array. But don't worry, Laravel 8.x has got your back with the AsArrayObject
cast!
To use this cast, all you have to do is change your cast from array
to AsArrayObject
in your model. It's as simple as that! Here's an example:
use Illuminate\Database\Eloquent\Casts\AsArrayObject;
class User extends Model
{
protected $casts = [
'metadata' => AsArrayObject::class
];
}
Once you've set this up, you can update specific properties on your JSON column without changing the entire array. This is super handy, especially if you're dealing with large amounts of data. Here's an example of how you can use it:
$user = User::find(1);
$user->metadata['notify'] = true;
$user->metadata['foo']['bar'] = 'baz';
$user->save();
It's important to note that AsArrayObject uses PHP's ArrayObject, which means that you can't use the usual array functions on these JSON columns. But don't worry, you can use Laravel's toArray() collection method if you need to access JSON in an array form.
So, if you want to update your JSON columns without any hassle, the AsArrayObject cast is the way to go! It's super easy to use and will make your Laravel application even more powerful. Give it a try and let us know how it goes!
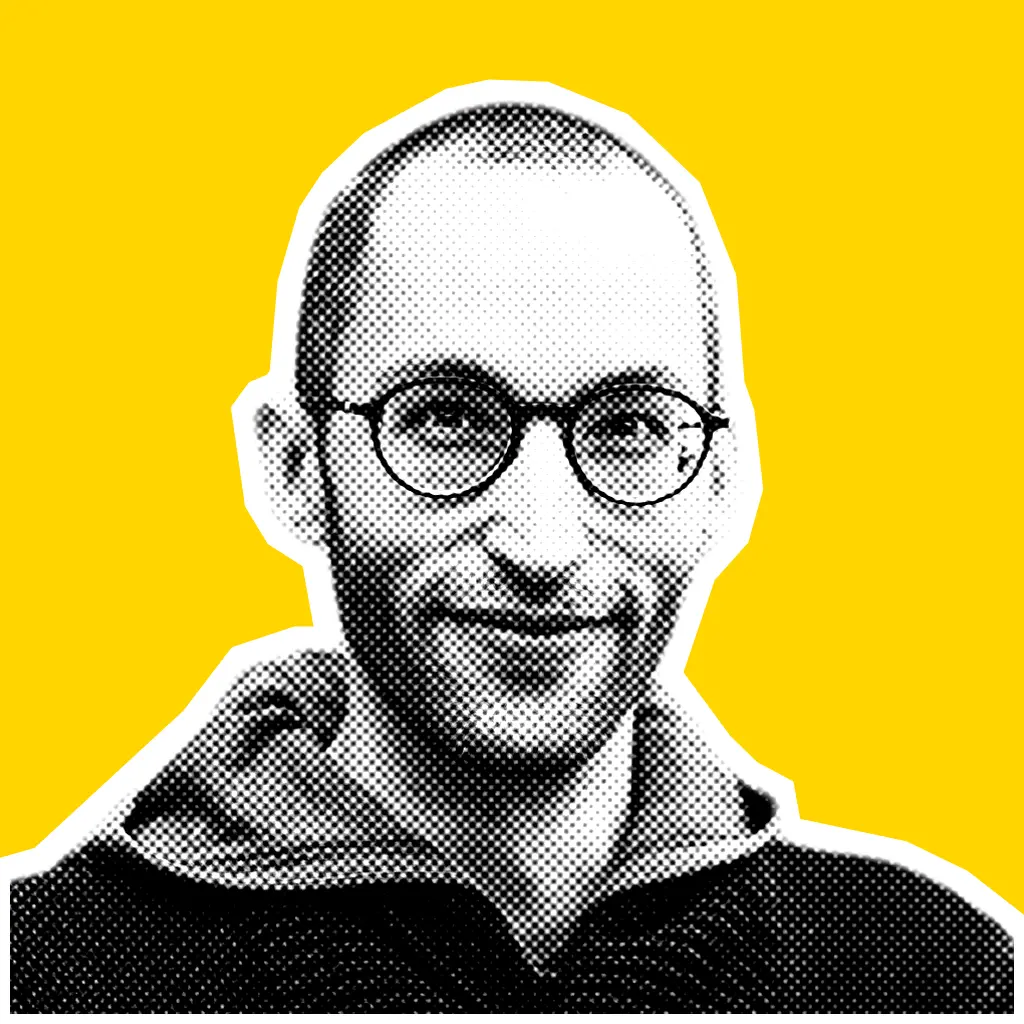
I'm a full-stack web developer working with the TALL stack.