PHP in_array Function
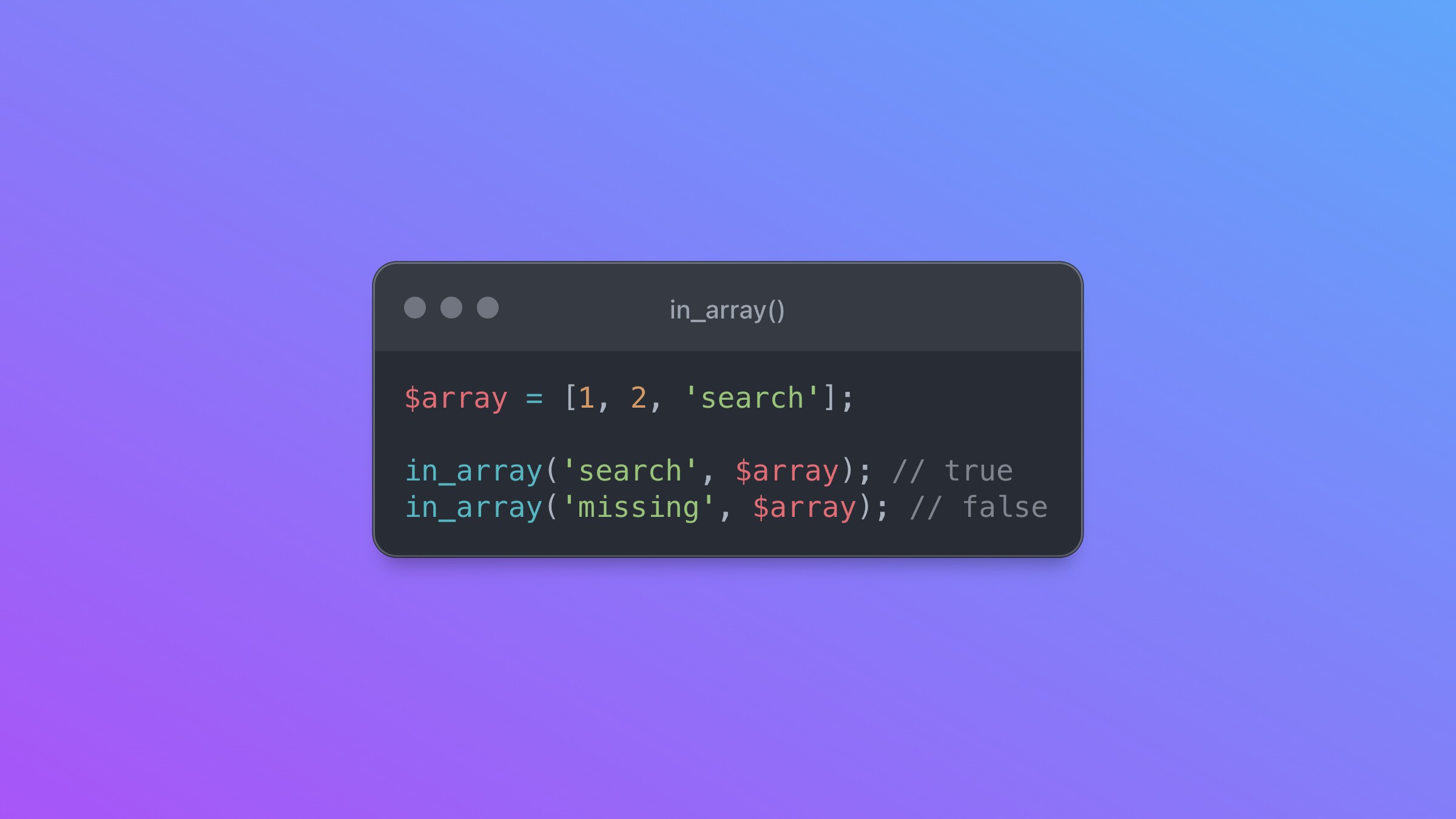
- Introduction to PHP's in_array() Function
- in_array() Without Strict Type Checking
- in_array() With Strict Type Checking
- Arrays on Stereoids With Laravel Collections
- Extend Laravels Array Helper
in_array()
Function
Introduction to PHP's The in_array()
function in PHP checks if a value exists in an array. It returns true
if the value is found, false
if not. The function is case-sensitive, distinguishing between uppercase and lowercase characters.
Basic syntax of in_array()
function:
bool in_array ( mixed $needle , array $haystack [, bool $strict = FALSE ] )
Parameter | Description |
---|---|
$needle |
The value to search for in the array. |
$haystack |
The array in which to search for the value. |
$strict |
[optional] - If set to true , the function will also check for the same type of the given value. By default, it is set to false . |
in_array()
Without Strict Type Checking
Search a string in an array of strings:
$array = [1, 2, 'search'];
in_array('search', $array); // true
in_array('missing', $array); // false
in_array()
With Strict Type Checking
Search an integer
in an array:
The third parameter true
will check for the type of your input:
$array = [1, 2, '3'];
in_array(3, $array, true); // false
in_array('3', $array, true); // true
Laravel Collections
Arrays on Stereoids WithFirst lets convert an array to a collection:
$array = ['apple', 'banana', 'orange'];
$collection = collect($array);
Now you can use the contains method to search your array:
$collection->contains('banana'); // true
Read more about collections in my Laravel Collection Tips and Tricks article.
Extend Laravels Array Helper
Add this to your AppServiceProvider
:
Arr::macro('contains', function($array, $needle) {
return in_array(
$needle, $array instanceof Collection
? $array->flatten()->toArray()
: static::flatten($array)
);
});
Now you can use it like so:
Arr::contains('search', $array);
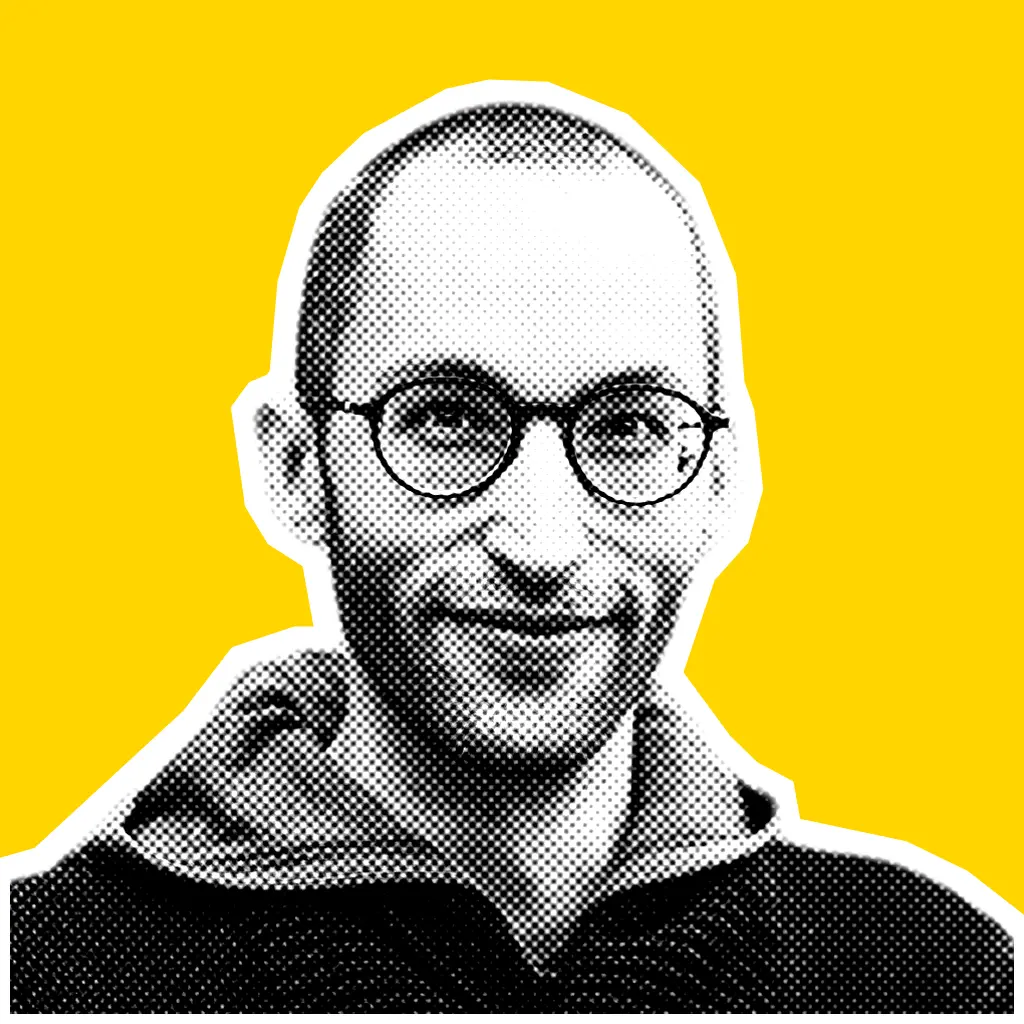
I'm a full-stack web developer working with the TALL stack.